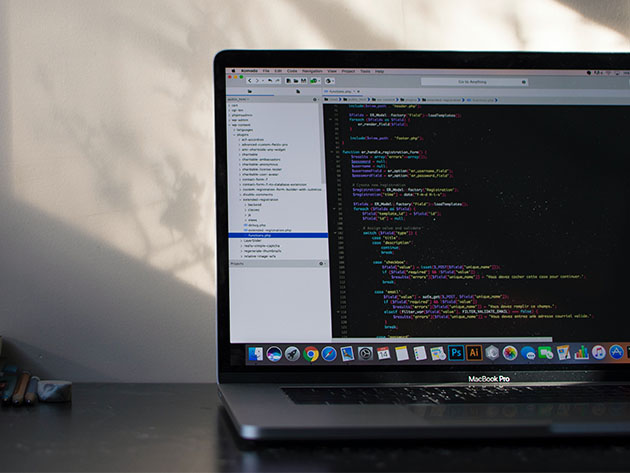
JavaScript Master Class Course
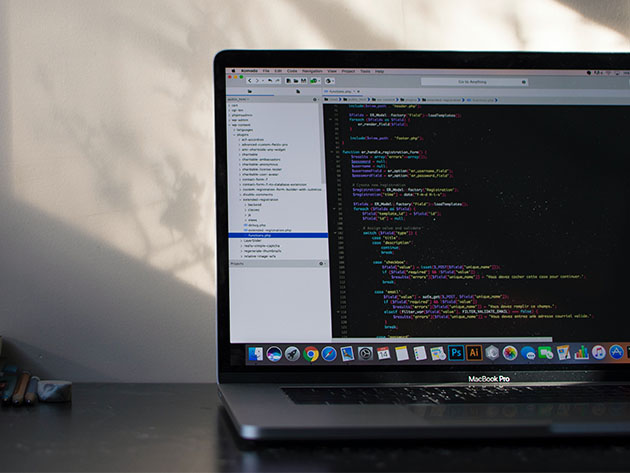
320 Lessons (34h)
- Your First Program
- JavaScript - The FoundationsVariables - Animation Intro4:53Variables Workspace4:20Variables Cheat SheetString - Animation Intro5:40String Workspace5:45Strings Cheat SheetOperators - Animation Intro7:48Operators Workspace7:06JavaScript Foundations Review17:02
- Launching a Career in TechGet Out of Tutorial HellLanding Your First Developer JobDealing with Time Management and Imposter SyndromeWhat to Put in a Junior Developer Portfolio
- JavaScript Foundations - Build Your First Mini AppsTemperature Gauge ChallengeTemperature Gauge Challenge Video Walkthrough9:51How Old Am I ChallengeHow Old Am I Challenge Video Walkthrough8:22
- Deep Dive into JavaScript ObjectsObjects - Animation Intro4:43Objects Workspace3:54Dot and Bracket Notation - Animation Intro5:12Dot and Bracket Notation Workspace4:40Object Constructor Function - Animation Intro6:26Object Constructor Function Workspace6:03The Secret Life of JavaScript Primitives7:23
- Handling Lists with JavaScript ArraysJavaScript Array Syntax - Animation Intro4:27JavaScript Array Syntax Workspace4:15Array Built In Methods Animation5:17Array Built In Methods Workspace4:19Array Methods Part 2 Animations Intro5:23Array Methods Part 2 Workspace6:54Array Review12:39
- Array Focused ProjectsUsing Arrays to Create Random InsultsUsing Arrays to Create Random Insults - Video Walkthrough9:21Mini Project: Random Insult Generator Video Walkthrough
- JavaScript FunctionHow to Write a Function - Animation Intro4:26How to Write a Function Workspace3:46Deeper Dive with Function - Animation Intro5:29Deeper Dive with Functions Workspace5:03Functions Part3 - Whiteboard5:15Functions Part3 - Lab7:02
- JavaScript Functions: Project SectionRock, Paper, Scissors Alternative GameRock, Paper, Scissors Alternative Game- Video Walkthrough18:25Rock, Paper, Scissors Alternative Game- Video Walkthrough - Step by Step10:12Rock, Paper, Scissors Alternative Game - CodeMini App: Hit the GymMini App: Hit the Gym - Video Walkthrough21:28Mini App: Hit the Gym - Step by Step7:50Hit the Gym - Code
- JavaScript Conditionals and LoopsLooping Around Part 1 - Whiteboard Animation7:54Looping Around Part 1 Workspace7:13Switch - Whiteboard3:51Switch - Lab7:36Loops Part2 - Whiteboard7:37Loops - Part2 - Lab7:11
- JavaScript Loops & Conditionals: Mini AppMagic 8 Ball9:12Magic 8 Ball - Video Walkthrough
- JavaScript Regular ExpressionsRegex8:48Regex Lab
- Context: This, Bind, Call & ApplyThis and Bind - Whiteboard5:04This and Bind - Lab5:59This and Call - Whiteboard5:57This and Call - Lab5:19
- Functional ProgrammingFunctional Programming Part 1 - Whiteboard9:03Functional Programming Part 1 - Lab8:29Functional Programming Part 2- Whiteboard7:26Functional Programming Part 2 - Lab7:09Functional Programming Part 3 - Whiteboard7:37Functional Programming Part 3 - Lab8:17
- Whiteboard AlgorithmsIdentify Unique String9:36Identify Longest Word in a String8:22Permutation of Two Strings9:34
- ES6Let Statements - Whiteboard5:29Let Statements Lab7:57Const Declaration - Whiteboard and lab3:08Arrow Functions - Whiteboard5:25Arrow Function - Lab6:13Spread Operator - Whiteboard6:15Spread Operator - Lab6:28
- More Algorithm ChallengesEnvironment SetupRepeat String with For Loop8:00Remove Odd Numers from Array Filter Method5:37Palindrome8:51Sum of Range6:45Repeat String with While Loop5:53Remove Elements from Head7:57Name Swap Indices8:44Remove Odd Number from Array with Modulus Operator and For Loops8:45Reverse a String11:57Reverse-a-String-Part-21:43Reverse-a-String-Part-35:31Reverse-a-String-Part-45:31Find-Longest-String-Part-112:05Find the Longest String Part 28:27Filter String Array12:00Is-Palindrom11:57
- Introduction to the DOMIntroduction-to-the-DOM7:51DOM-Tree-Nodes6:07
- Todo AppTodo-List-Project-Overview4:42Todo-List Document.querySelector()-Document.getElementById()8:37Todo-List Changing-the-DOM-with-textContent6:11Todo-List More-DOM-Methods-and-Properties10:07Todo-List User-Interactions-and-Event-Listeners4:10Todo-App-Without-Local-Storage13:41Todo-List Working-with-Forms6:25Todo-App Local-Storage6:10Todo-App-With-Local-Storage13:38CSS3:09How-to-Share-Code2:23
- Matching GameMatching-Intro6:14Matching-Part-19:41Matching-Part-22:27Matching-Part-32:07Matching-Part-44:19Matching-Part-52:33Match-Part-68:15Matching-Part-73:35Matching-Part-82:18Matching-Part-95:13
- Quote MachineQuote Machine IntroQuote-Machine-HTML-CSS-Video-Walkthrough13:30Quote Machine JavascriptQuote-Machine-JavaScript-Video-Walkthrough16:20Quote-Machine-Deploy1:37
- HTML & CSS PrimerWebsite-Overview1:50Website-Text-Editor2:35HTML-Intro6:14HTML-Elements-and-Tags6:56HTML-About3:45HTML-Services8:42HTML-Comments-and-Structure7:06HTML-Header6:01HTML-Hero4:28HTML-Testimonal6:40HTML-Footer4:09CSS-Intro5:32CSS-Header9:34CSS-Hero5:14CSS-Internal-Navigation4:04CSS-About-and-Testimonial3:03CSS-Footer2:46CSS-Services2:47
- Front End CookbookCSS-Animations-Video-Walkthrough15:39CSS-Animations-Challenge-Video-Walkthrough15:02CSS-Transitions-Video-Walkthrough9:22CSS-Transitions-Challenge-Video-Walkthrough9:27JS-Debugger-Part34:49JS-Debugging-Part16:06JS-Debuggin-Part25:02JS-Moment-Basics8:32JS-Moment-Christmas-Countdown11:46
- ReactReact-From-Scratch12:09Challege-Using-JSX8:49Code-Refactor3:01Rendering-One-Element3:54React-Babel7:07React-Rendering-Multiple-Elements4:20Creating-Stateless-Functional-Components7:04Stateless-Functional-Components-Practice4:17Creating-Class-Components6:16Class-Components-Practice3:27Styling-Stateless-Functional-Components8:04Styling-Class-Componets8:36Styling-Within-Component9:29Complex-Components6:10Setting-Up-Project6:11Building-Our-Components3:50Styling-Our-Components-Part16:39Styling-Our-Components-Part25:30Props-Part17:10Props-Part25:51Props-Part37:48Codepen-Challenge13:36Codepen-Challenge22:30Codepen-Challege35:25Codepen-Challenge43:17Codpen-Challenges5Props-Project10:05Iterating-Through-Lists10:10Iterating-Through-Lists-Refactor1:59Rendering-Two-Filtered-and-Transformed-Lists-to-the-DOM7:15Filter-and-Map-On-Array4:20Codepen-Challenge-Part14:32Codepen-Challenge-Part24:20Color-Spectrum-Refactor6:55Friendly-Filter-and-Map-Two-Lists-DOM6:54React-Events-Part28:35Useless-Note-Taker-Introduction-to-Events10:14Guess-My-Age-Intro-to-State13:36Food-Menu-Vote13:50React-Ajax-Requests18:45React-Forms10:52Friendly-App-Refactor-with-Map7:16
- Svelt - Build Apps with Svelt.jsInstructor NoteSvelte Info1:21Section Requirements3:34Text Editor Setup1:59
- Svelte Project - Budget CalculatorIntro2:46Starter Application4:40Folder Structure8:51Setup FilesAdd Global CSS & Font Awesome3:02Component Overview4:45Navbar Component Intro5:43Navbar Component7:17Title Component3:32Props Basics9:43CSS9:32Each Block9:48Expenses Data4:04Expenses Component11:49Else and Passing Props12:56Expense Component4:24If Block3:51Events9:46Component communication6:55Props Drilling9:14SetContext and GetContext10:02createEventDispatcher9:52Clear Expenses Button4:53Reactivity15:07Form Setup7:57Two Way Binding7:43Empty Values Functionality12:18Form Submission5:18Add New Expense6:28setModifiedExpense11:04Pass Edit Values into Form13:01Edit Expense6:1634.5-other-option13:15Toggle Form7:33Lifecycle Functions5:50Setup Local Storage API10:05afterUpdate6:39Slot Basics10:30Complete Modal4:32Transition Basics8:36Transition Parameters3:35Transition - in: and out:1:55Modal Transitions2:28Simple Expense Transition6:44Key Expression in Each Block and Animate9:26HTTP Request Using onMount14:57HTTP Request using #Await Blocks6:50Deploy on Netlify - Drag and Drop3:44Deploy on Netlify - Continuous Deployment5:38
- Svelte Project - Ecommerce App - RazorInstructor NoteProject DemonstrationIntro13:19Intro13:19Setup FilesBootstrap Svelte Application3:22Folder Structure and Resources5:22Setup Project Pages7:27Svelte Router Setup9:06Url Parameters4:52Hero Component10:29Local Data Structure3:59Svelte Store Benefits/Basics5:32Products Store Setup11:23Flatten Products5:11Store Unsubscribe2:45Store Unsubscribe Shorthand2:20Products Component Complete5:36Products Component Complete7:45Loading Component4:46Featured Component6:43Derived Stores7:15Single Product Page11:41svelt:head element3:18Small Navbar9:35Cart Button3:27Big Navbar5:00Links4:36Toggle Navbars5:32Basic Sidebar7:01Global Store Basics8:16Global Store Method4:46Setup Close Sidebar Function5:46Sidebar Transitions1:45Cart Basics6:18Cart Structure11:36Cart Store Initial Setup7:10Cart Items8:16Single Cart Item6:50Cart List Transitions2:49Cart Total5:32Remove Item7:43Increase Amount8:44Decrease Amount4:56Decrease Amount Refactor - Optional1:14Add to Cart9:05LocalStorage Setup6:32User Store Setup4:20Login/Logout Links9:47Checklist5:13Strapi Info1:39Bootstrap Strapi App4:17Strapi Basics3:15Products Content Type4:16Add Products3:59API Access5:30getProducts4:27Products Store8:51Image Problem Fix4:21Login Page - Variables4:49Login Page - HTML13:23Login Page - Basic Functionality8:32Login Page - General Overview6:13registerUser Function11:17loginUser Function6:10User Store UpdateUser Store Modifications6:30setupUser Function10:56navigate5:06Alert Basics7:20Configure Alert10:54Alert with Form Submissions4:53Close Alert Programmatically2:15Double Check Login Functionality2:41Checkout Page Overview0:55Checkout Page Basics2:19Restrict Access2:48Empty Cart2:43Checkout Form - Basic Setup6:50Setup Stripe Account4:21Stripe Elements - HTML7:37Stripe Elements - JavaScript8:52Stripe Token5:08Empty Cart Error1:43Order Content Type3:53Submit Order Function12:39Complete Submit Order13:07Complete App7:40Free Cloudinary Account1:58Connect Cloudinary with Strapi7:05Free Heroku Account1:49Install Heroku CLI2:31Deploy Strapi on Heroku13:22Setup Backend5:46Deploy Svelte APP on Netlify2:20
A Comprehensive 34-Hour Course Just About Every Fact on JavaScript You Could Ever Hope to Know
Rob Merrill is a Front End Engineer who enjoys working with JavaScript. He lives in Seattle with his wife, cat and two dogs in an apartment that is too small to hold them all. He is an App Specialist at Subsplash in Seattle. He started coding around two years ago, meaning he knows some stuff but he also knows what it means to know nothing.
Description
If you want to land your first developer job then JavaScript is a great way to break into the industry with high in-demand skill. This course will guide you from step one in developing this coding superpower! This course is built to solve your problems! Both up to date with the newest JavaScript features this course is also timeless in teaching the essentials that everyone needs to know. Each minute of this course was created with the goal of helping you become a great engineer!
- Access 320 lectures & 34 hours of content 24/7
- Master the basics of the language, variables, objects, arrays, & functions
- Understand how to design the structure of the code you write
- Leverage Javascript's built-in methods to increase your productivity regardless of what libraries or frameworks you use
- Develop practical skills around higher-order functions that you will utilize for years to come
- Build awesome projects to fill your personal portfolio
"Engaging presenter. Good mix of theory with ample review and exercises to reinforce the learning. Excellent option for someone with little java experience and minmal programming experience in general." – Andrew Gregory Garton
Specs
Important Details
- Length of time users can access this course: lifetime
- Access options: desktop & mobile
- Certificate of completion included
- Redemption deadline: redeem your code within 30 days of purchase
- Updates included
- Experience level required: beginner
- Have questions on how digital purchases work? Learn more here
Requirements
- Any device with basic specifications
Terms
- Unredeemed licenses can be returned for store credit within 30 days of purchase. Once your license is redeemed, all sales are final.