The 2024 Java & Spring Bundle
What's Included
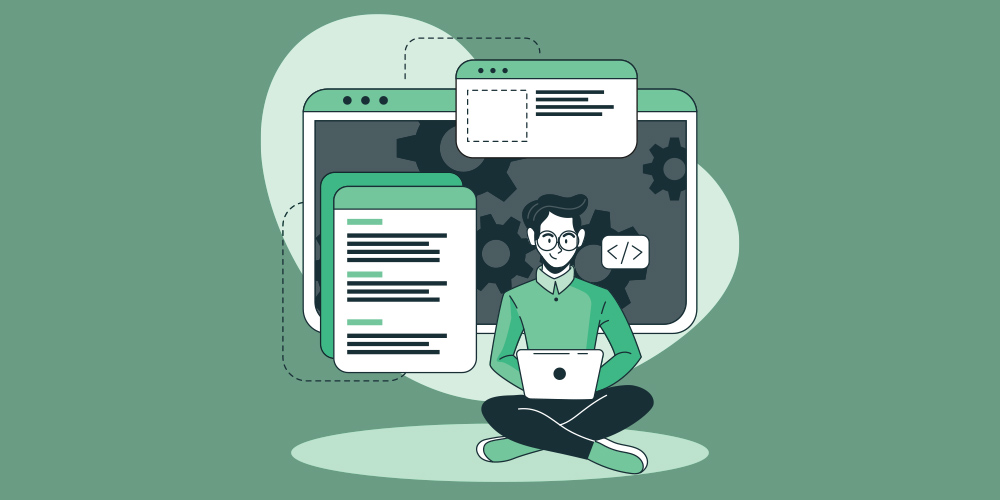
Java Programming: Learn Core Java & Improve Java Skills
- Experience level required: All levels
- Access 140 lectures & 20 hours of content 24/7
- Length of time users can access this course: Lifetime
Course Curriculum
140 Lessons (20h)
Your First Program
Introduction
What We Will Learn In This Java Course?2:51Environment Setup
Getting Started With Java? ( JDK, JRE and JVM )4:54Installing Java on Windows8:19Installing Java on Linux4:27Installing Java on Mac5:29Development Environment
What is IDE?2:15Download and Run Eclipse7:16First Java Program - Hello World4:42Compiling and Running Applications0:55Closer Look at the First Java Program1:48Basics of Java Syntax
What Are Variables?10:20Closer Look at the Java3:10Basic Output13:24Basic Input5:01Comments10:32Data Types8:30Hexadecimal Octal and Binary Data Types16:32Type Conversion & Type Casting5:41Stack & Heap1:38Arrays7:35Operators
Arithmetic Operators5:05Assignment Operators5:42Unary Operators5:33Equality and Relational Operators4:37Conditional Operators4:55Bitwise and Bitshift Operators23:14Char Data Type13:56Operator Precedence4:13Expressions, Statements and Blocks3:00Control Flow Statements
If, If-Else Statement5:34If-Else - If Ladder Statement, Nested If Statement10:13Switch-Case Statement7:04Project 1: Calculator8:29For Loops6:44For-Each Loop6:12While Loop12:51Infinite Loop17:28Do - While Loop16:16Break Keyword4:45Continue Keyword2:37Return Keyword3:09Labeled Loops10:46Project 2: Fibonacci Series7:18Methods ( Functions )
What is Method?3:42Method Calling1:14Types of Methods & Method Return Types9:07Java Var-Args17:17Method Overloading3:36Project 3: Find Exponential Number5:30Java Object Oriented Concept
Object & Class6:01Stack & Heap7:48Access Modifiers13:41Naming Conventions2:20Constructors21:16Packages23:37Constructors10:31Packages11:39“Static” Keyword8:38Static import13:12Nested & Inner Classes16:09Local inner classes12:47Object Oriented Programming
Section Overview1:11Inheritance23:08Sealed Class9:47Method Overriding7:43“Super” and “This” Keywords8:28“Final” Keyword7:26Abstract Classes10:05Interfaces13:16Polymorphism7:16Encapsulation9:03Anonymous Class10:43Wrapper Classes, Auto-Boxing and Unboxing
What is Wrapper Class in Java?1:35Conversion of Types1:52Autoboxing - UnBoxing4:15Strings
Section Overview1:10What is String?14:19Equality of Strings29:22Immutability of Strings9:12Useful Methods of String - Part 113:34Useful Methods of String - Part 217:05Why String is Immutable?1:37StringBuffer Class5:35StringBuilder Class3:30String vs String Buffer vs String Builder1:43Project 4: Reverse String3:55Collections
Introduction to Collections10:44Section Overview1:08List Interface13:23ArrayList Class17:17Conversion Between Lists and Arrays17:06Sorting Array List13:22Comparator11:57Searching ArrayList8:51Itrator and ListIterator15:41Set Interface - Part 113:41Set Interface - Part 213:03Queue12:54Deque8:13Map Interface - Part 111:55Map Interface - Part 212:28Exception Handling
Intro to Exception2:01What Is The Difference Between Error and Exception?3:15Exception Types2:43Try – Catch Block1:58Finally Block12:34Differences Between "Throw" and "Throws"1:00Throws Keyword5:39Exception Methods6:41Project 5: Bank Account Balance14:40Java Desktop Application Design (Swing)
Introduction to Java Swing2:25Throw Keyword4:44Swing Containers, Labels, Text Fields and Buttons2:30Layouts7:19Project 6: Login Form in Swing16:56Enum Types
Enum Types8:47Project 7: Traffic Lights Program With Enum6:59Lambda Expression
Marker and Functional Interfaces3:20Section Overview0:42Lambda Expression9:58Predicate15:13Project 8: Calculator app with Lambda10:34Date&Time
Section Overview0:45Calendar Class20:45Local Date Class16:24Local Time Class11:35Local Date Time Class11:44Period Class9:30Date Time Formatting16:28Java I/O
Introduction to Java I/O4:35Section Overview1:07OutputStream Class18:49InputStream Class - Part 18:06InputStream Class - Part 216:18Writer Class13:38Reader Class21:19Project 9: Copying a file with Java I/O9:51Object Serialization and Deserialization19:17transient keyword10:35What We Have Learned ?
What We Have Learned ?6:51
Java Programming: Learn Core Java & Improve Java Skills
Oak Academy | Long Live Tech Knowledge
Oak Academy is a group of tech experts who have been in the sector for years and years. Deeply rooted in the tech world, they know that the tech industry's biggest problem is the "tech skills gap" and their online course is their solution. They specialize in critical areas like cybersecurity, coding, IT, game development, app monetization, and mobile. Thanks to their practical alignment, they are able to constantly translate industry insights into the most in-demand and up-to-date courses.
Description
Learn by Doing with 7 Projects and 50+ Coding Tasks
This Java course takes you from absolute beginner core concepts, like showing you the free tools you need to download and install, to writing your very first Java program. You will learn the core Java skills step by step with hands-on examples. If you are from the field and need a refresher this course will be a guide for you, too. Every time you come back to this course you will learn something new or improve yourself.
What you will learn
- Access to 140 lectures & 20 hours of content 24/7
- Understand what Java is & how it operates
- Acquire programming fundamentals using Java
- Gain proficiency in using the Eclipse IDE
- Learn about the Java Development Kit (JDK)
- Understand the Java Runtime Environment (JRE)
- Comprehend the role of the Java Virtual Machine (JVM)
- Master all concepts of Object-Oriented Programming
- Use the 'static' keyword & work with nested and inner classes
- Understand the concepts of superclasses & subclasses
- Manipulate strings effectively in Java
- Work with wrapper classes for primitive data types
- Handle exceptions using 'throw' & 'throws' keywords
- Develop graphical user interfaces with Swing
- Design layouts using different Grid Layouts
- Understand and use Enum types in Java
Who this course is for
- Beginners with no coding experience who want to learn Java, including Java 11 and Selenium WebDriver.
- Programmers aiming to enhance their Java skills to an expert level
- Those wanting to master core Java concepts like Strings, Exceptions, Swing, and Enum Types
- Individuals interested in building various types of applications, including desktop, web, enterprise, and mobile apps, as well as games with Java
- Career changers seeking to become Java developers
- Aspiring computer programmers interested in creating visually appealing applications and learning game and Android app development
Specs
Important Details
- Length of time users can access this course: lifetime
- Access options: desktop & mobile
- Redemption deadline: redeem your code within 30 days of purchase
- Experience level required: all levels
- Certificate of Completion ONLY
-
Closed captioning is NOT available
-
NOT downloadable for offline viewing
- Have questions on how digital purchases work? Learn more here
Requirements
- Basic computer knowledge
- Computer with 64-bit operating system
- Windows OS is preferred but not mandatory
- Eclipse IDE is preferred
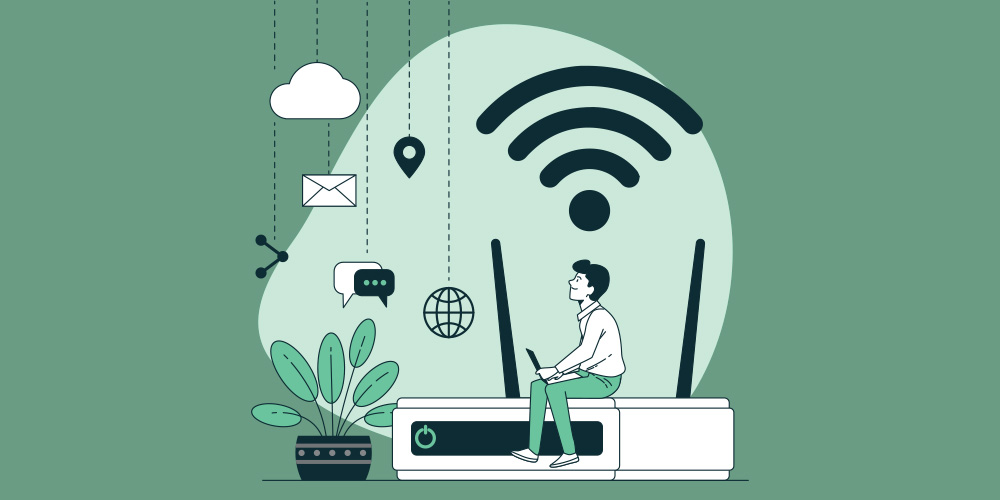
PostgreSQL | Learn T-SQL Using PostgreSQL with Real Examples
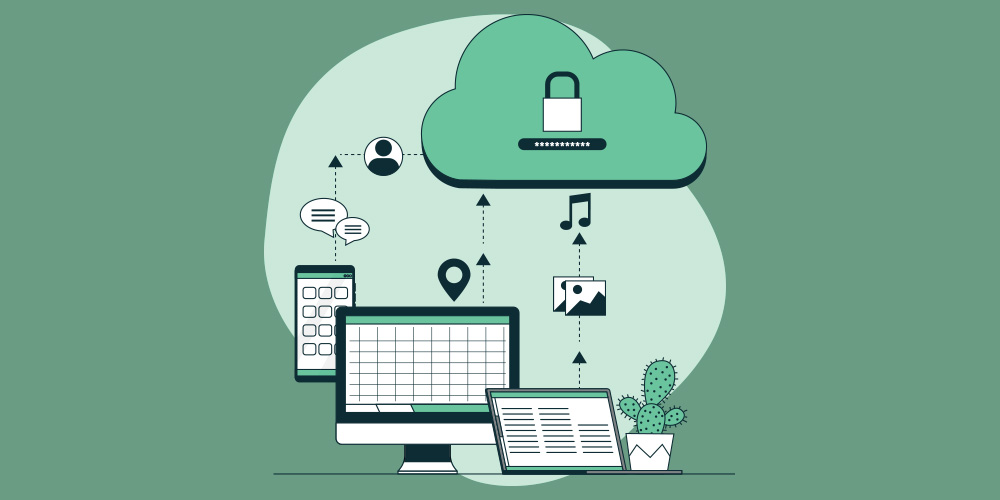
Microservices | Spring Cloud Microservice with Rest API
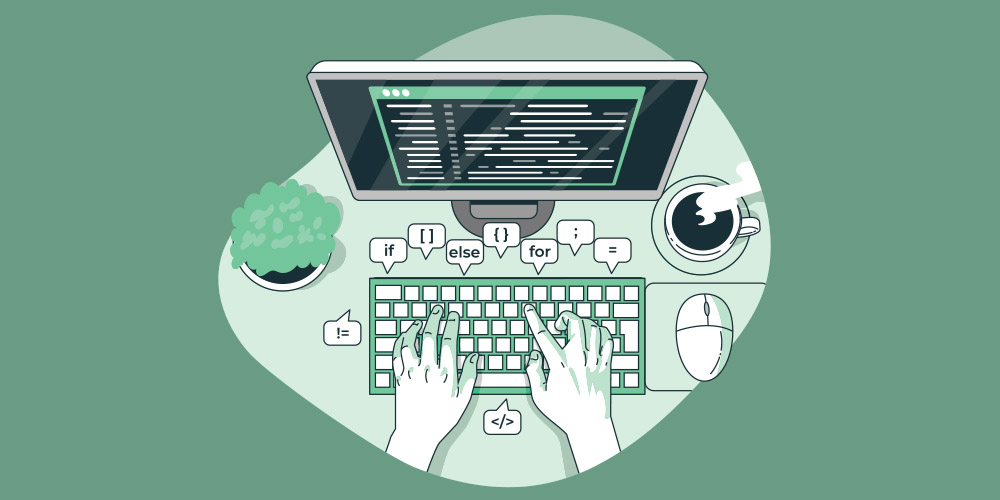
Complete Git & Github Beginner to Expert
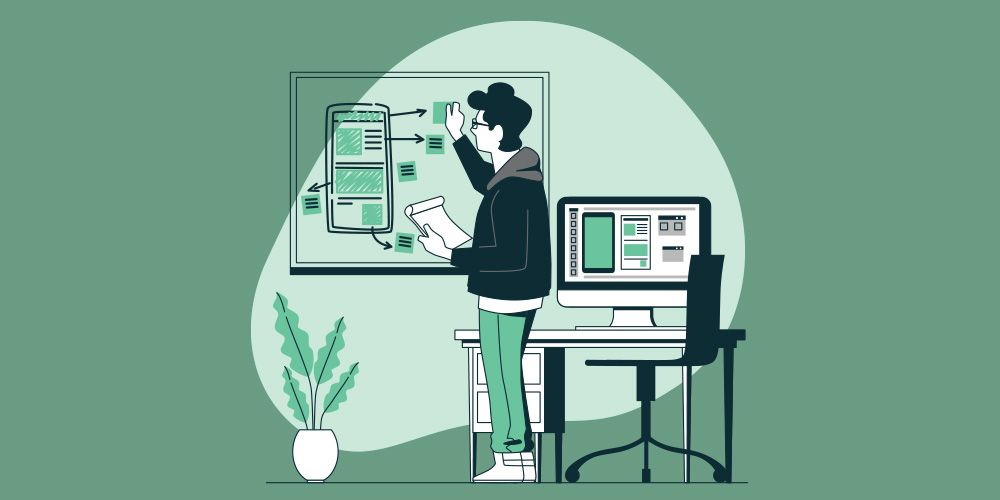
Spring Framework | Spring Boot For Beginners with MVC, Rest
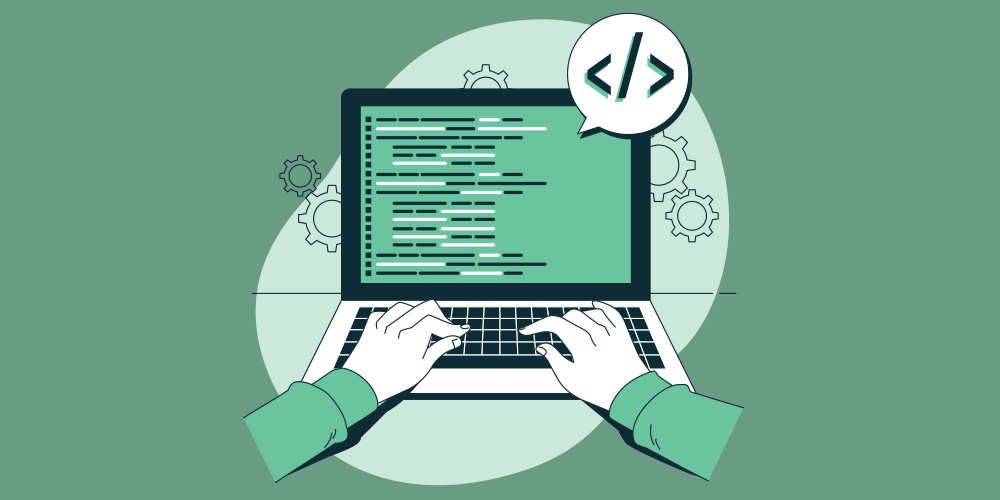
JPA | Learn Spring Boot, Spring Data JPA & Hibernate Basics
Terms
- Unredeemed licenses can be returned for store credit within 30 days of purchase. Once your license is redeemed, all sales are final.